Simplifying Authentication in React and Node.js: OAuth2 vs. Clerk
Aug 19, 2024
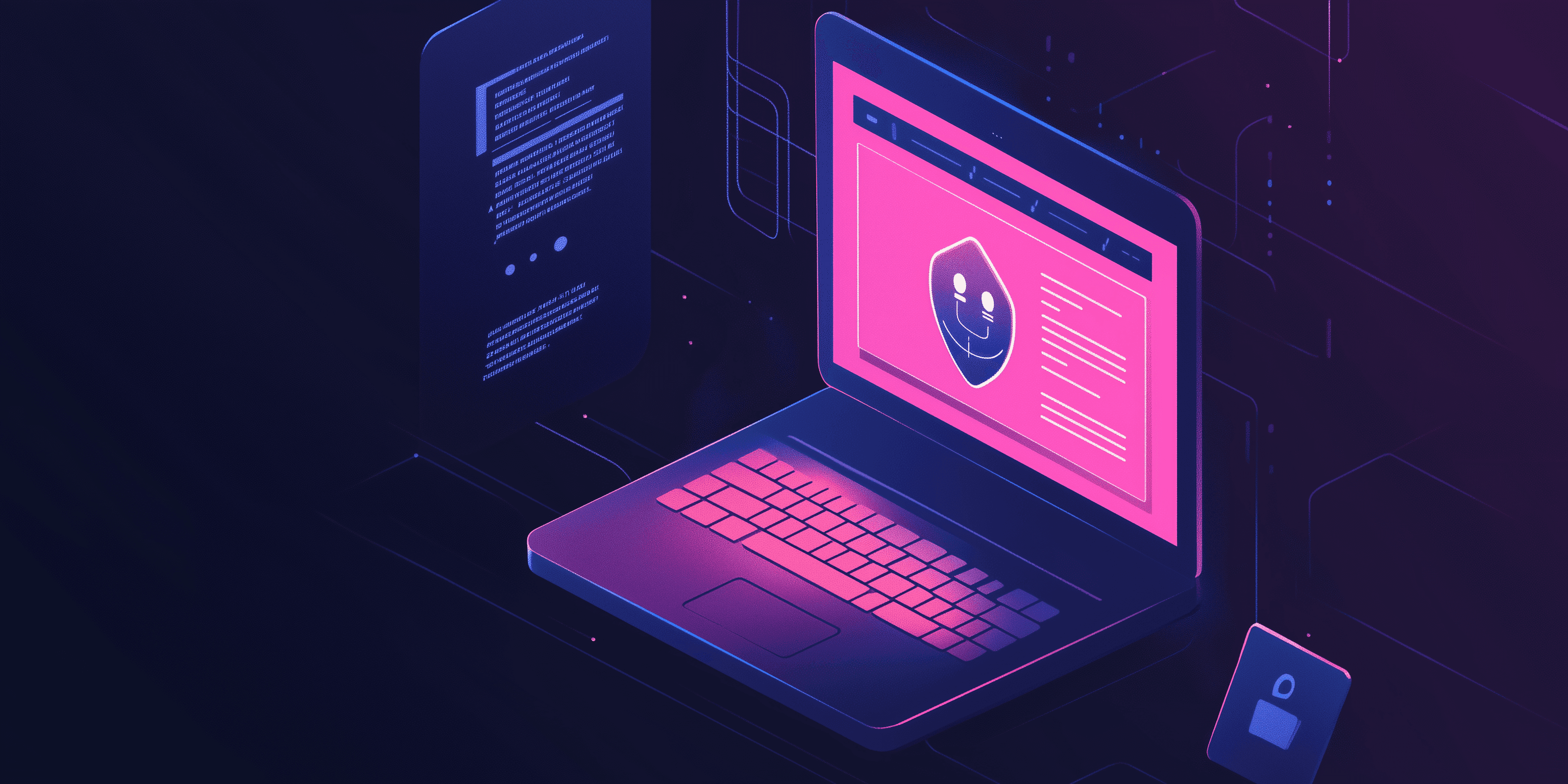
When building modern web applications, handling user authentication is crucial. Traditionally, OAuth2 has been the go-to protocol for implementing secure authentication and authorization flows in React and Node.js apps. However, newer solutions like Clerk have emerged, simplifying this process even further. In this blog post, we’ll explore the basic differences between implementing OAuth2 and Clerk in a React frontend and Node.js backend setup. By the end, you’ll understand how Clerk can streamline your authentication process, making it easier to manage user sessions and permissions.
Understanding OAuth2 Implementation
OAuth2 is a widely adopted protocol for authorizing users via third-party services like Google or GitHub. Here’s how it typically works when implemented in a React frontend and Node.js backend:
React Frontend with OAuth2:
User Redirect: The user initiates the login process, which redirects them to an OAuth2 authorization server.
Authorization Code: After a successful login, the authorization server redirects back to your app with an authorization code.
Token Exchange: The frontend sends this authorization code to your Node.js backend to exchange it for an access token.
Node.js Backend with OAuth2:
Access Token Request: The backend makes a POST request to the OAuth2 provider, exchanging the authorization code for an access token (and sometimes a refresh token).
Token Storage: The backend securely stores these tokens and uses them to make authorized API requests on behalf of the user.
This process works well, but it requires careful management of tokens, including refreshing them when they expire, securing them, and implementing logic to check permissions.
Clerk: A Simpler Way to Handle Authentication
Clerk simplifies the authentication process by offering pre-built components and SDKs that handle much of the complexity for you. Here’s how Clerk compares to OAuth2 in a React and Node.js setup:
React Frontend with Clerk:
Pre-Built Components: Clerk provides pre-built sign-in, sign-up, and session management components, making it easy to integrate authentication into your app.
Automatic Session Handling: After a user signs in, Clerk manages the session automatically, storing session information locally and making it accessible via Clerk’s frontend SDK.
Node.js Backend with Clerk:
Session Verification: Clerk offers a Node.js SDK that simplifies verifying user sessions. When a request is made from the frontend, Clerk’s middleware can verify the session token (usually a JWT) passed in the request.
Abstraction of Token Management: Unlike OAuth2, where you handle tokens manually, Clerk abstracts this process, making it more seamless.
Access Tokens and Authorization Headers:
OAuth2: Generates access tokens that are typically sent via the Authorization header as Bearer <token>.
Clerk: Clerk also generates tokens, but they are managed internally by Clerk’s SDK. You can send these tokens via the Authorization header, similar to OAuth2, but with less manual handling required.
Implementing Scopes and Permissions with Clerk
While OAuth2 uses scopes to define what a token can access, Clerk uses a more straightforward approach with roles or user metadata. You can implement role-based access control using Clerk’s middleware in your Express.js app:
In this example, Clerk’s withAuth() middleware verifies the session, and you can add custom logic to check for specific roles or permissions.
Conclusion
While OAuth2 remains a robust solution for handling authentication and authorization, Clerk offers a more streamlined and user-friendly approach. By abstracting much of the complexity and providing pre-built components, Clerk allows you to focus on building your application rather than managing tokens and permissions. If you’re starting a new project or looking to simplify your existing authentication flow, Clerk is definitely worth considering.
With Clerk, you can achieve secure and efficient authentication without the overhead of manual token management, making it an excellent choice for modern web development. I am currently in the process of implementing Clerk in my new project which uses Next.js as a frontend and Node.js as backend for it's API. I am, of course, dogfooding Project Etherite for this new project which is called Gatemap. I explained a little about it in my recent post about implementing force-directed graphs in D3.js. But stay tuned (or check subsequent posts if you're from the future) for more information about this project, which I'll start sharing as it gets closer to completion.