Building Force-Directed Graphs in React with D3.js
Aug 12, 2024
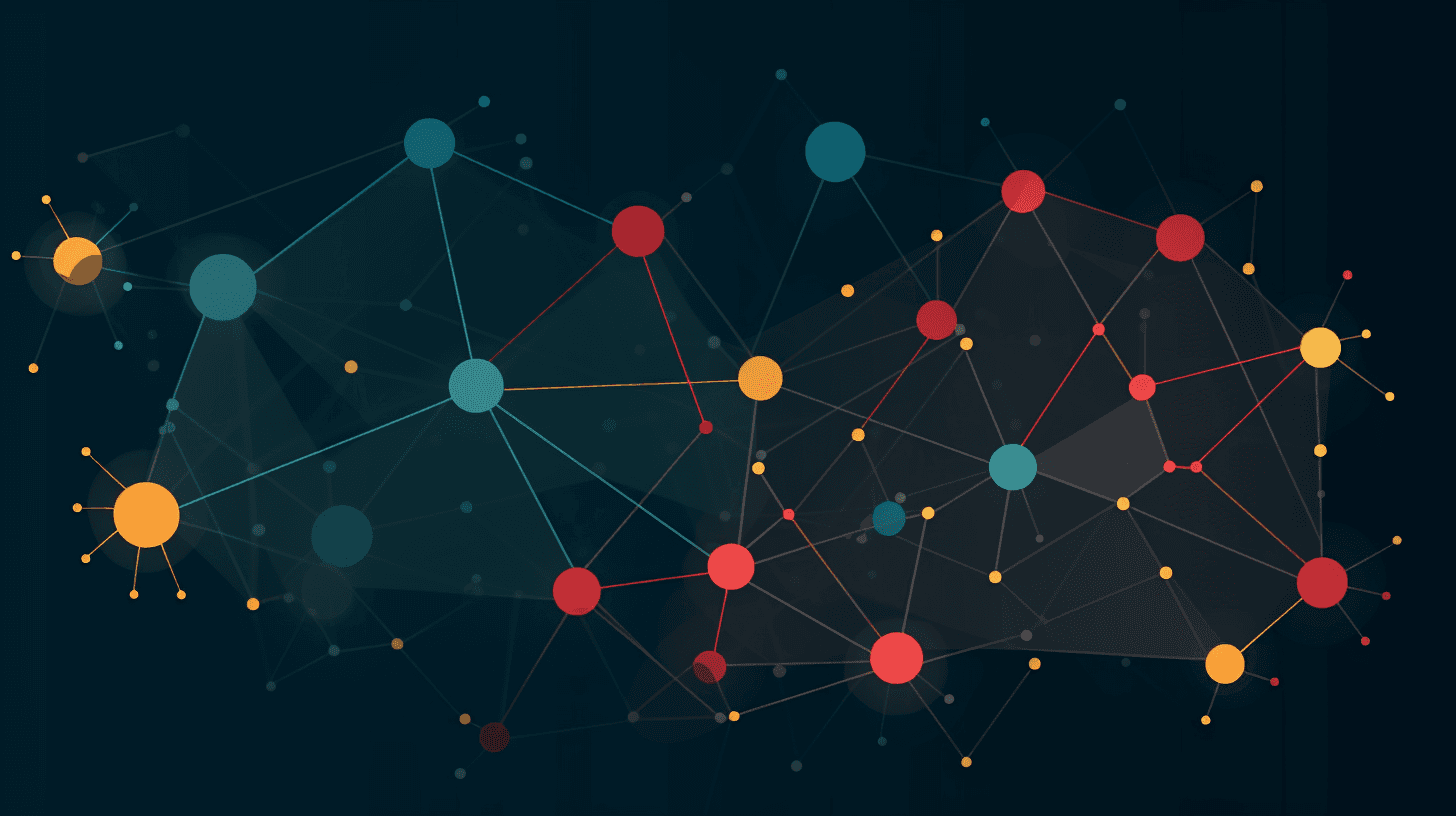
Introduction
Force-directed graphs are an intriguing and visually appealing way to represent complex data relationships. These graphs, often used in network visualizations, utilize algorithms that simulate physical forces to determine the positions of nodes and the lengths of edges, creating a layout that naturally separates connected components while clustering related nodes together.
In this article, we will explore what force-directed graphs are, why they are useful, and how you can implement them in a React application using D3.js, a powerful JavaScript library for data visualization.
What is a Force-Directed Graph?
A force-directed graph is a type of graph layout that positions nodes in a way that minimizes overlap and evenly distributes them based on their connections. The layout algorithm applies forces akin to physical forces: nodes repel each other like magnets, while edges act like springs that pull connected nodes together. This results in a balanced and aesthetically pleasing visualization of complex networks.
Key Components:
Nodes: Represent entities or data points.
Edges (Links): Represent the relationships or connections between nodes.
Forces: The physical-like forces that dictate the positioning of nodes and the length of edges.
Use Cases of Force-Directed Graphs
Force-directed graphs are often used in the following scenarios:
Social Network Analysis: Visualizing relationships between individuals or groups.
Biological Networks: Representing protein interactions or genetic relationships.
Knowledge Graphs: Showing connections between concepts or topics.
Web Crawlers: Mapping out website structures.
I am currently working on a project called Gatemap that implements force-directed graphs and allows users to map routes between different locations connected via portals in an online game called Albion Online. I'll make a detailed post about this project soon. Check back soon (or check later posts if you're from the future) if you're interested.
Implementing a Force-Directed Graph in React Using D3.js
Now that we have an understanding of what force-directed graphs are, let’s dive into implementing one in a React application. We’ll be using D3.js for the graph layout and rendering. I realize that there are component libraries for React with D3.js built-in which make it easier to implement common visualizations. However, understanding how D3.js works and how to implement can allow for far more flexibility when implementing complex visualizations.
1. Setting Up Your React Project
First, of course, we have to make sure that we have a React environment set up. If you don’t have a project ready, you can create one using Create React App:
Then, we install D3.js:
2. Creating the Graph Component
Next, we create a ForceGraph
component where we’ll build the graph.
Code Breakdown
The
ForceGraph
component takes in two props:nodes
andlinks
, which represent the graph’s nodes and the links (or edges) between them.
useRef
is used to create a reference (svgRef) to the SVG element, which allows direct manipulation of the DOM element.useEffect
is used to run the D3-related code after the component has mounted. This ensures the D3 code is run after the DOM elements have been rendered.
The
d3.select
function selects the SVG element referenced by svgRef. The width and height of the SVG are set to 800 and 600, respectively.
d3.forceSimulation(nodes)
initializes a simulation with the nodes..force('link', ...)
adds a link force, which positions the nodes based on the links between them. The distance of 100 determines how far apart linked nodes are..force('charge', ...)
adds a repulsive force between nodes (strength(-400) makes nodes repel each other strongly)..
force('center', ...)
centers the simulation in the middle of the SVG (width / 2, height / 2).
svg.selectAll('.link')
selects all elements with the class link (which is initially none)..data(links)
binds the links data to these elements..join('line')
creates new <line> elements for each link, applying the class link, stroke color#999
, and stroke width of 2.
svg.selectAll('.node')
selects all elements with the class node..data(nodes)
binds the nodes data to these elements..join('circle')
creates new <circle> elements for each node, applying the class node, radius 10, and fill color#69b3a2
..call(d3.drag()...)
attaches drag behaviors to the nodes, enabling dragging functionality.
The
tick
function is called on each iteration of the simulation.It updates the positions of the links (x1, y1, x2, y2 attributes) and nodes (cx, cy attributes) based on the current simulation state.
dragStarted: Called when dragging starts. It temporarily “fixes” the node’s position (d.fx, d.fy) and restarts the simulation with higher alpha (alphaTarget(0.3)).
dragged: Called while dragging, continuously updates the node’s fixed position (d.fx, d.fy) to follow the mouse.
dragEnded: Called when dragging ends, releasing the fixed position of the node (d.fx, d.fy set to null) and reducing the alpha back to 0 to stop the simulation.
The component returns an empty
<svg>
element that will be populated with nodes and links by D3.js. The ref attribute allows the svgRef to reference this element.
3. Preparing the Data
To visualize a graph, we need a set of nodes and links. Here’s an example of how we might structure this data:
We can now pass this mock data to the ForceGraph component:
4. Styling and Enhancements
We can enhance the graph’s appearance by adding styles, labels, or even animations. For example, we can adjust node colors based on their properties or animate the graph layout to create a dynamic visualization.
Customizing Node Appearance:
Adding Labels:
Conclusion
Force-directed graphs are a powerful tool for visualizing complex networks, and D3.js provides a robust foundation for implementing them in web applications. By combining React with D3.js, you can create interactive and dynamic visualizations that make data exploration more intuitive.
Hopefully, this guide has helped you understand the basics of setting up a force-directed graph in React using D3.js. From here, you can expand on this example by customizing the graph’s appearance, adding interactivity, or integrating it with real-world data sources. The possibilities are vast, and with a bit of creativity, you can create visualizations that truly bring your data to life.